Xogot Jam #1 Theme Reveal: “You’re holding it wrong”
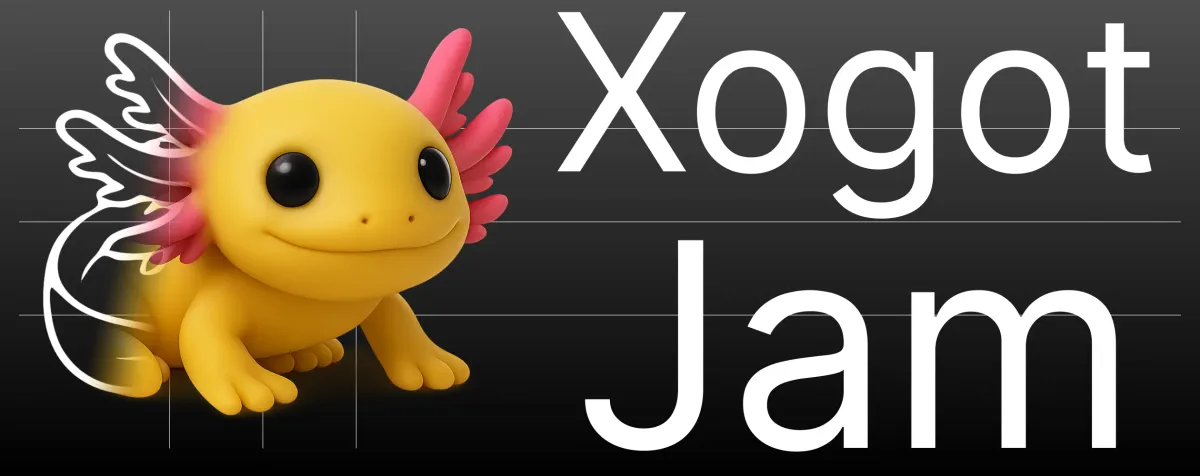
We’re excited to kick off the very first Xogot Game Jam — and the theme is:
"You’re holding it wrong"
Whether you choose to interpret it literally, metaphorically, or ironically, we can’t wait to see what you come up with. Games can explore orientation, misunderstanding, user error, or anything else this phrase inspires.
Jam Details
If you haven't already, you can still join the Xogot Jam:
- Join here: https://itch.io/jam/xogot-jam
- Jam ends: Sunday, June 29, 2025 @ 11:59PM EDT
- Discord: Join us in the Xogot Discord, where we’ve created a
#game-jam
channel for participants to chat, ask questions, and show progress
Free Xogot Access
Everyone who joins the jam can receive a free 1-month subscription to Xogot to use during the event.
Join the Xogot Jam and then claim free access to Xogot here.
Happy jamming!